
In a recent blog post & video, we showed you how to install Botpress v12 using the Docker Playground. Play with Docker is only an appetizer for what Docker and Botpress v12 could achieve together! For today’s blog, I will show you how to use a few basic - but very useful! - Botpress elements.
- How to use an Action in Botpress to call an API
- How to render default content in an action
- Understanding the default structure of a built-in element in Botpress
I made videos of this too! Click here to watch the first video that accompanies this blog, and the second part is out
What’s an Action?
A Botpress Action is a piece of code that Botpress v12can use in a flow. By default, the Axios and other libraries are bundled in a Botpress action. The action I’m going to create will use an API call to the GIPHY endpoint, through which my Botpress chatbot will retrieve gifs to share with its user.
An aside: Botpress Actions & Botpress Hooks are very similar. Since I want the Botpress conversation flow to trigger the retrieval, I’m going to use an action. Hooks are more commonly used to execute codes at specific points in the chatbot lifecycle.
GIPHY API
Before creating the Botpress code, you need to get a valid API Giphy key. This key can be found on the GIPHY developer website. You start by creating a Giphy App.

You’ll need to sign up for a Giphy account, or log in if you already have one.

On the main page, create an application and select the right hand API choice.
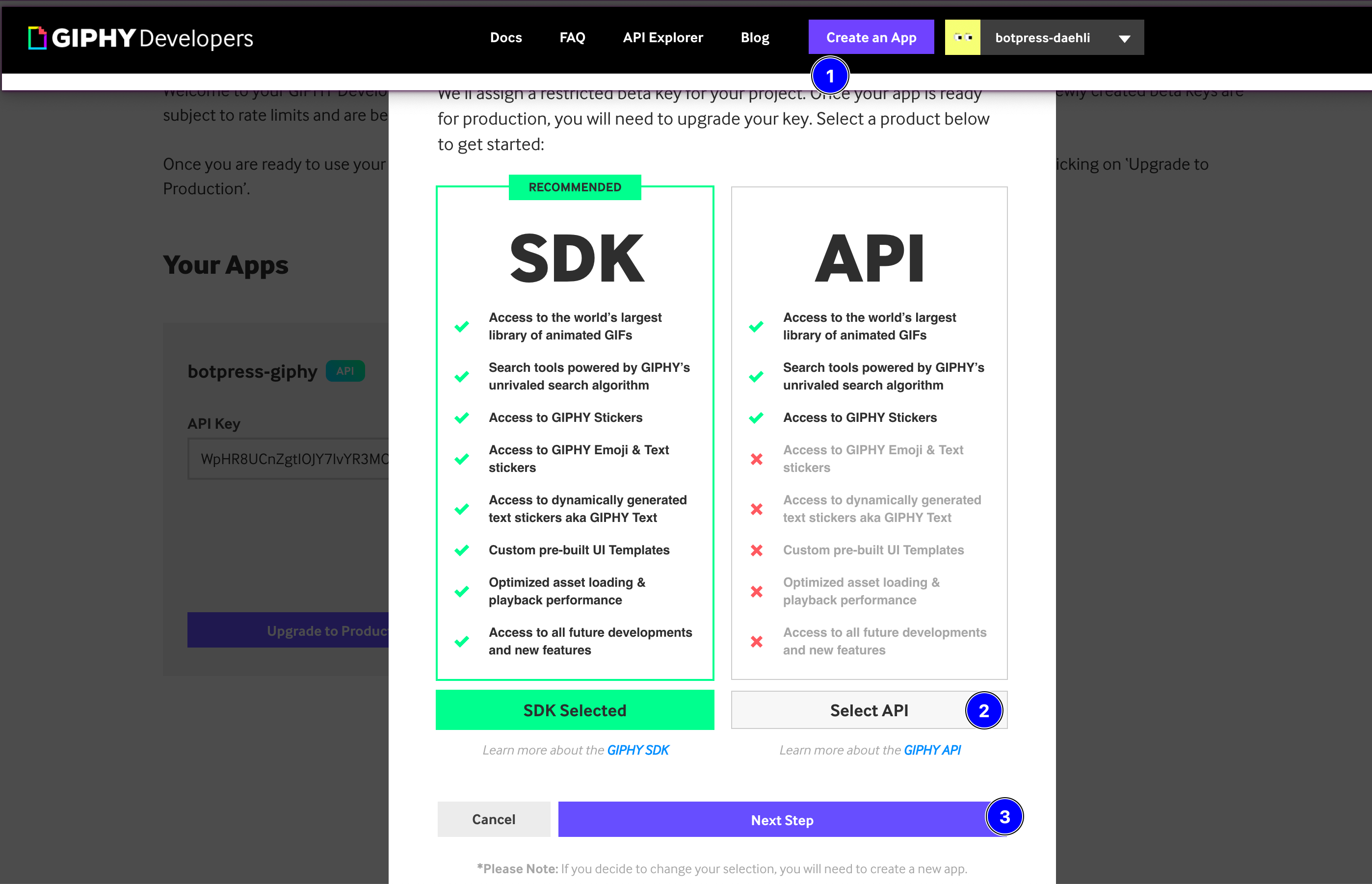
Yes, from the right hand side - the one that’s not recommended.
The SDK version is definitely an option, but you will need to do an extra step in Botpress - the SDK library needs to be injected into the action component. We’ll cover injecting an external library into Botpress in a future blog!
Next, give the app a name and a description.
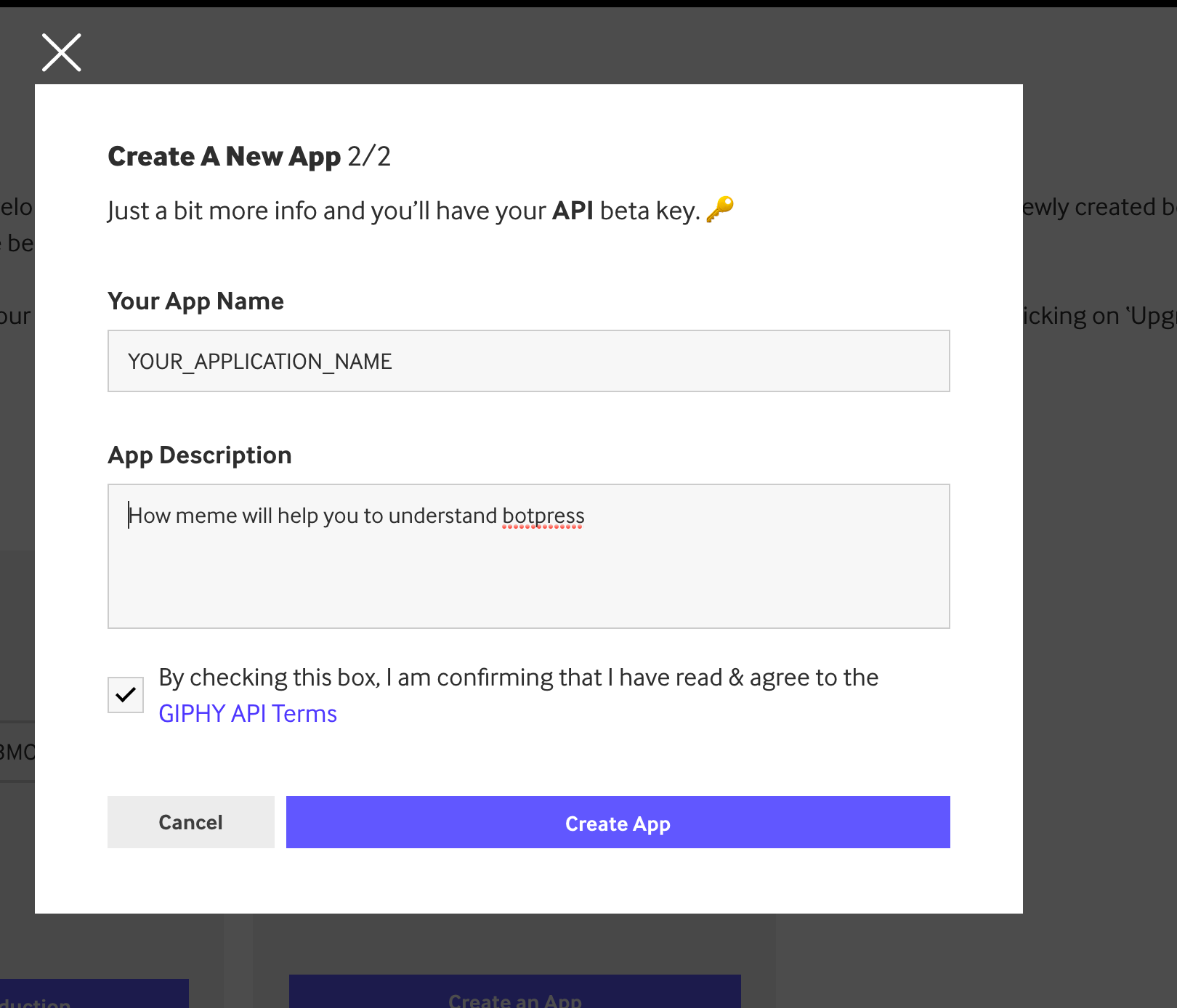
On the next screen, GIPHY will give you the API key that was created - hold onto it! You’ll be using this key in your Botpress chatbot.
It’s time to open Botpress!
Starting Botpress
There are a few ways to start Botpress v12:
If you’re running Botpress locally, from the source:
yarn run start
Or from the binary file
./bp
- Or from Docker.
docker-compose up -d
Once in Botpress, we will add the API key and the new actions to a bot. You can use the bot you created in our last blog, or create one from scratch.
At this point, you can add your API key from GIPHY.
Using an Environment & Setting up your API Key
You need to go in your chatbot - I named my chatbot giphy. Once in your chatbot, go in the Code Editor > Configurations > bot.config.json. At the end of the file add your API key, with the name of the chatbot and the variable apiKey:
"giphy": { "apiKey": "DQHOmOjCRpGvFCQ9lY46C3gPTZzEq77Q"}
Now you can use this environment in your action. More on that later, but the idea here is that the variable botConfig will contain all the variables from your bot.config.json. The giphy.js action that you're going to create in the next step will define & use that variable, and the first parameter of mergeBotConfig is the name of your bot (in this case, giphy).
Create the Action
Now let’s add the actions that will make the chatbot work. In the left side panel, go in the Code Editor `</>`, choose the Actions tab, and click on the +. The first action I’m going to create is called giphy.js, then I click `Submit.` This action will contain the code to call the Giphy API and return an image based on the search query you pass to it.
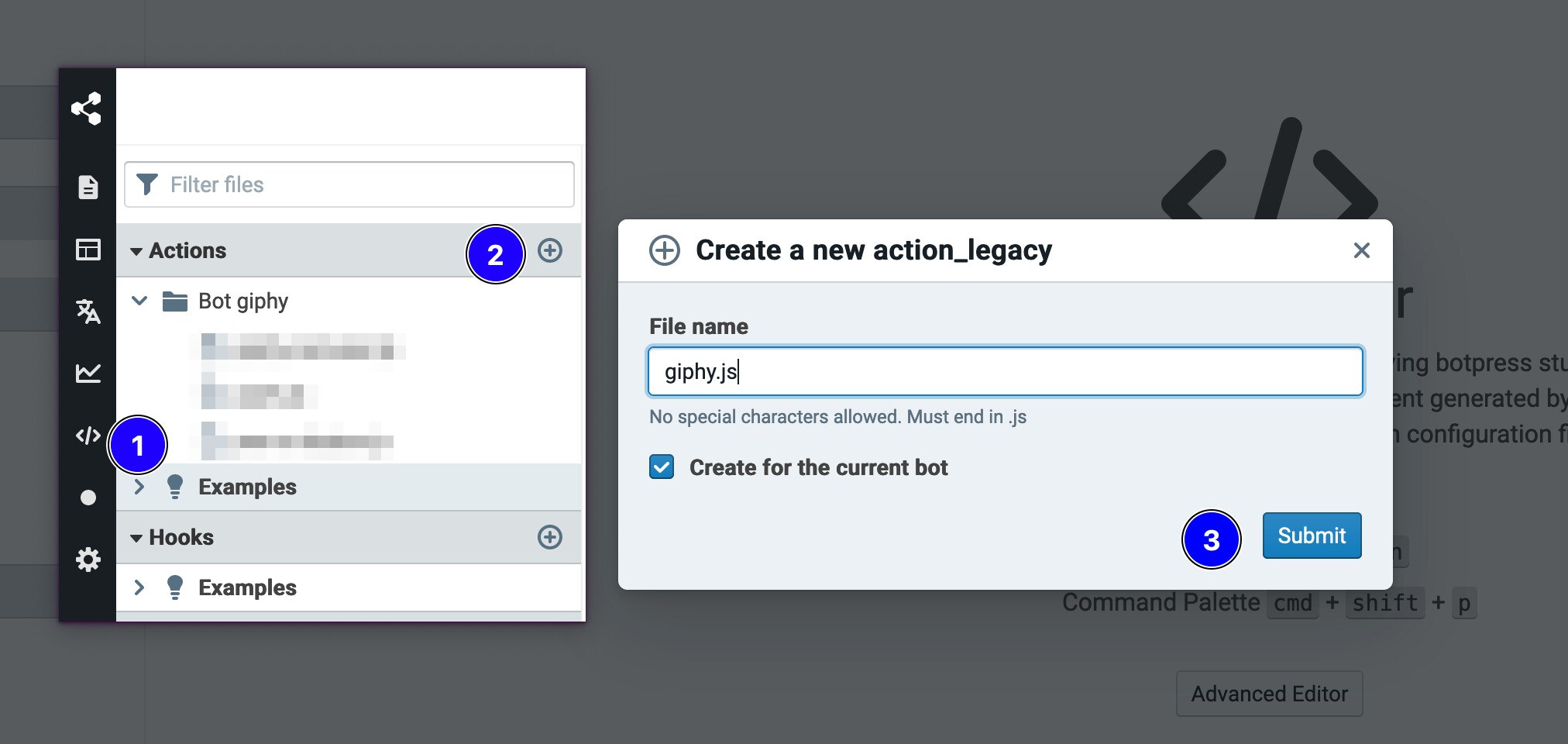
Here is the code that I wrote for my giphy.js action - to call the giphy.com API and return the image.
```jsx
function action(bp: typeof sdk, event: sdk.IO.IncomingEvent, args: any, { user, temp, session } = event.state) { /** Your code starts below */ const axios = require('axios') /** * Fetch one GIF image * @title Get image from Giphy * @category Example * @author Botpress */ const giphy = async () => { const regex = /!giphy/i let parsedText = event.payload.text.replace(regex, '') if (parsedText == '' || parsedText.toLowerCase().trim() == 'help') { parsedText = 'Help : `!giphy `' const payloads = await bp.cms.renderElement('builtin_text', { text: parsedText, markdown: true }, event) return await bp.events.replyToEvent(event, payloads) } // const apiKey = await bp.ghost.forBot('giphy').readFileAsObject('/', 'bot.config.json') const botConfig = await bp.config.mergeBotConfig('giphy', {}) const response = await axios.get( `https://api.giphy.com/v1/gifs/search?q=${parsedText}&api_key=${botConfig.giphy.apiKey}` ) if (response.data.data.length === 0) { parsedText = `Didn't find any picture for the following Query **${parsedText}**` const payloads = await bp.cms.renderElement('builtin_text', { text: parsedText, markdown: true }, event) return await bp.events.replyToEvent(event, payloads) } const payloads = await bp.cms.renderElement( 'builtin_image', { image: response.data.data[0]['images']['original']['url'], title: response.data.data[0]['title'] }, event ) return await bp.events.replyToEvent(event, payloads) } return giphy() /** Your code ends here */}
```
Create the Nodes & Transitions in Botpress
In the flow editor, create two new nodes and a new Transition.
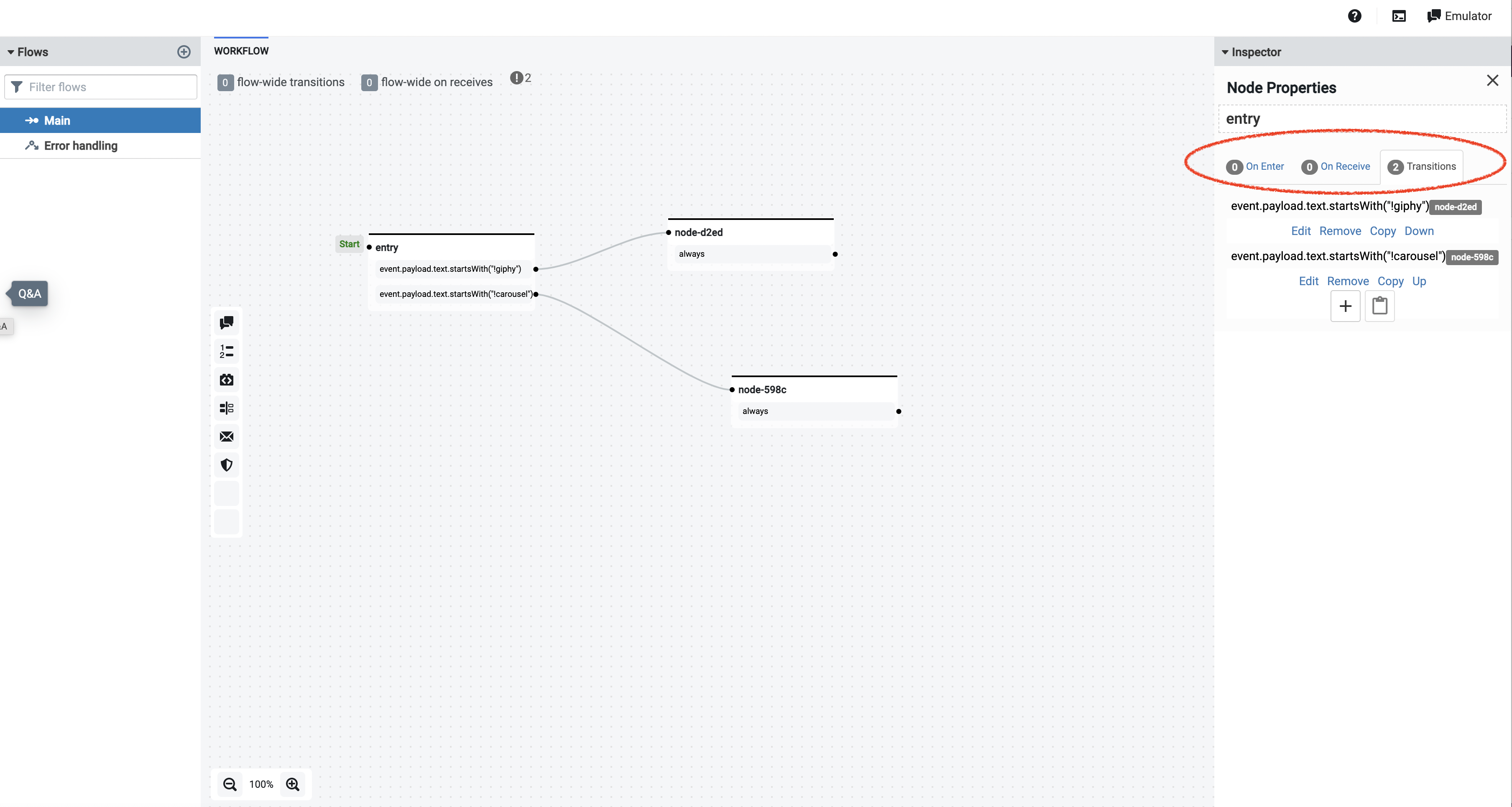
In entry node with the #1 label, I’m using a raw text statement as a command.
(Don't worry about the second transition or the third node - we’ll update those later in this blog.)
event.payload.text.startsWith("!giphy")
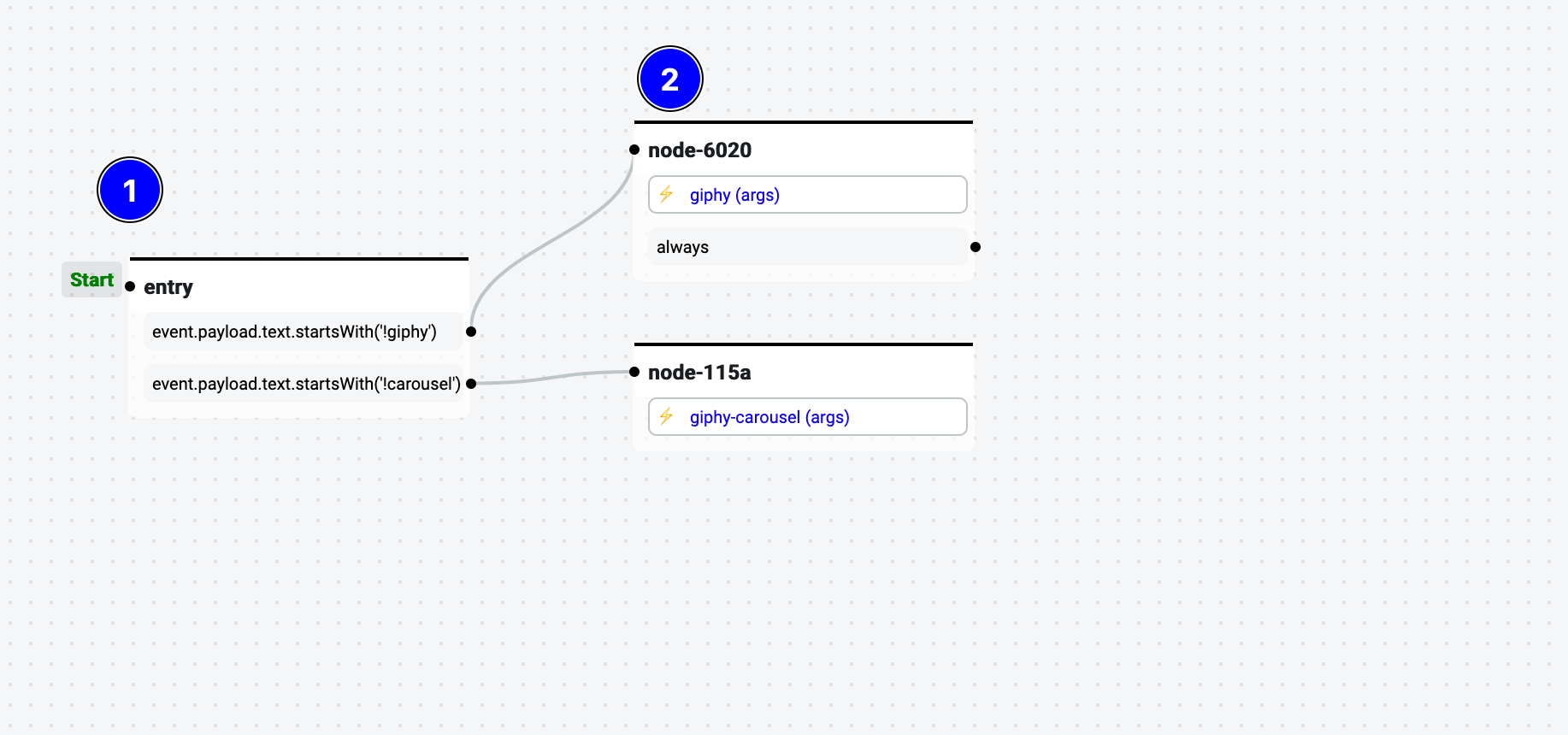
Create the Action to Render the Carousel
Returning a giphy on its fine on its own, but we can make it more powerful. We can use a carousel component to do that. Botpress’s carousel component can be used to display lots of useful things to chatbot users - individual t-shirts from an e-commerce site, items on a menu from a restaurant, vehicles to rent, etc. In this bot, I’m going to display images from the search query sent to the Giphy API.
Once again, I will create a new action, this one called Carousel.js. This action will display 5 images returned from Giphy when we send the user’s search query, and give the user the option to choose. Follow the same process as above to create a new action, and include this code:
function action(bp: typeof sdk, event: sdk.IO.IncomingEvent, args: any, { user, temp, session } = event.state) { /** Your code starts below */ const axios = require('axios') /** * Giphy Carousel * @title Created A giphy Carousel * @category Custom * @author Botpress */ const carousel = async () => { const regex = /!carousel/i let parsedText = event.payload.text.replace(regex, '') if (parsedText == '' || parsedText.toLowerCase().trim() == 'help') { parsedText = 'Help : `!carousel <Picture to search>`' const payloads = await bp.cms.renderElement('builtin_text', { text: parsedText, markdown: true }, event) return await bp.events.replyToEvent(event, payloads) } const botConfig = await bp.config.mergeBotConfig('giphy', {}) const response = await axios.get( `https://api.giphy.com/v1/gifs/search?q=${parsedText}&api_key=${botConfig.giphy.apiKey}` ) let cards = [] for (let i = 0; i < 5; i++) { // If the response is empty don't push the value if (response.data.data[i]) cards.push({ title: response.data.data[i]['title'], subtitle: response.data.data[i]['subtitle'], image: response.data.data[i]['images']['original']['url'], actions: [ { action: 'Open URL', url: response.data.data[i]['images']['original']['url'], title: response.data.data[i]['title'] } ] }) } console.log(cards) const payloads = await bp.cms.renderElement( 'builtin_carousel', { items: cards }, event ) return await bp.events.replyToEvent(event, payloads) } return carousel() /** Your code ends here */}
After you create the carousel action, you can update the nodes in your flow editor to recognize the carousel command.
event.payload.text.startsWith("!carousel")
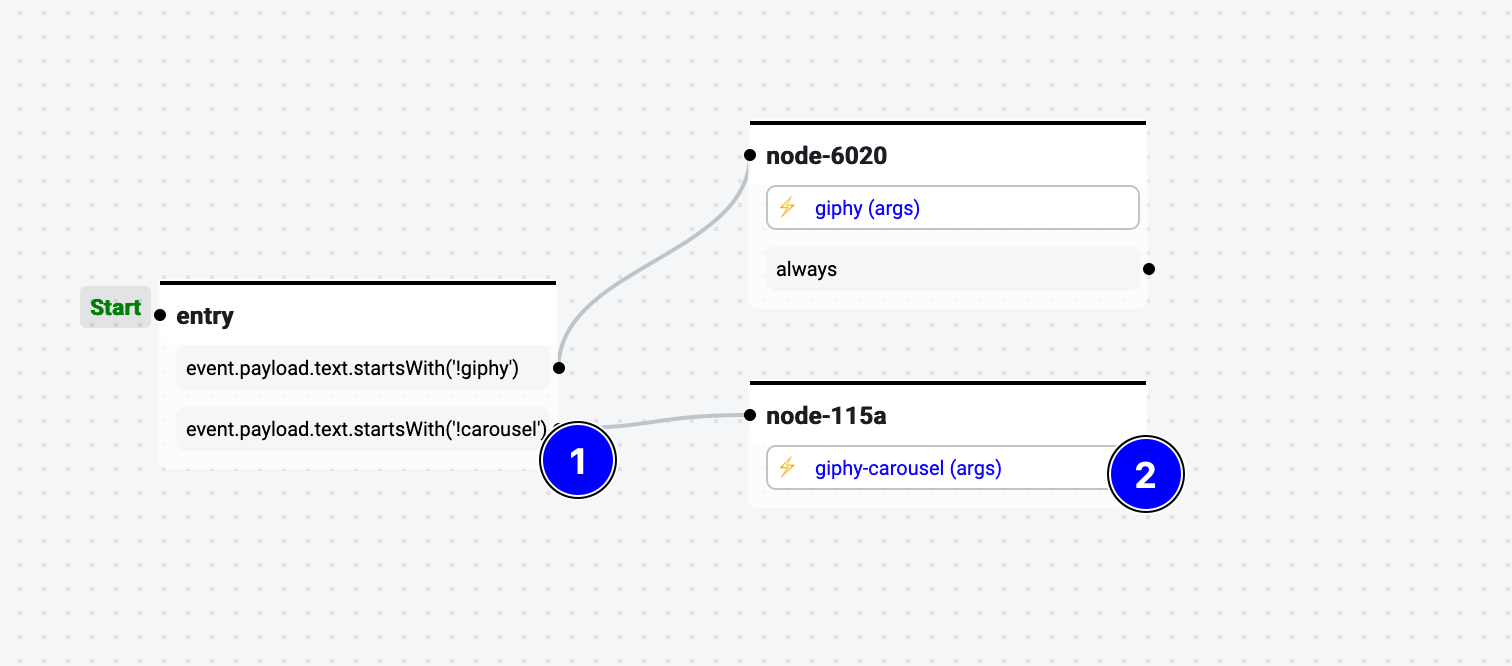
Using your New Giphy Chatbot
And you’re done! Now your bot can understand and act on just two commands:
- !giphy <subject>
- !carousel <subject>
Here's what I got when I said !giphy dog to my bot:

And here's what I got when I said !carousel cat to my bot:
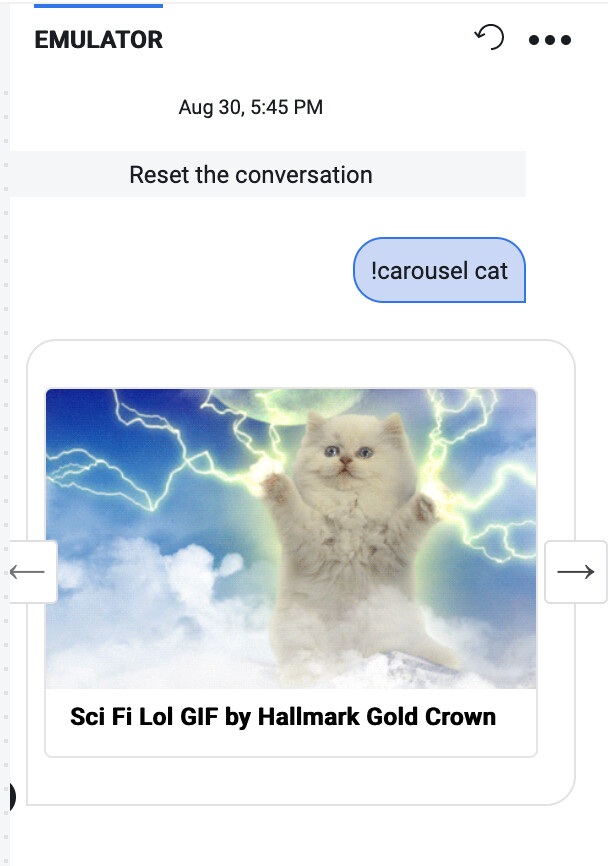
Next Steps & Further Learning
I hope this tutorial helped you! If you’re interested, please head over and join our Botpress community, where you can pose questions and learn from other Botpress developers.
If you want more information on what we covered today, here’s a list of resources for further exploration:
- Botpress docs on creating actions
- Docs on calling an external API from Botpress
- Overview of the Botpress v12 Platform, particularly the developer experience (video)
And if you like this content, sign up for the Botpress newsletter!
Table of Contents
Stay up to date with the latest on AI chatbots
Share this on: