Webchat Client
The JavaScript client enables seamless interaction with the Webchat API directly from your web application. Specifically designed for browser environments, this client allows you to send and receive messages from the bot and subscribe to various events, such as incoming messages.
This is for advanced use cases where you want to build your own front-end or listen to particular events.
We recommend most people start with the Embedded Webchat or React Components.
Quickstart
1. Install the package
Install the necessary package from the npm public registry.
npm install @botpress/webchat
pnpm add @botpress/webchat
yarn add @botpress/webchat
2. Obtain the Client ID
To integrate Botpress Webchat Client into your application, you need to obtain your bot's Client ID, which uniquely identifies your bot and enables communication with the Webchat service. Follow these steps to retrieve it:
- Open your bot in the Botpress Workspace
- Navigate to the Webchat tab
- Access Advanced Settings
- Copy the Client ID
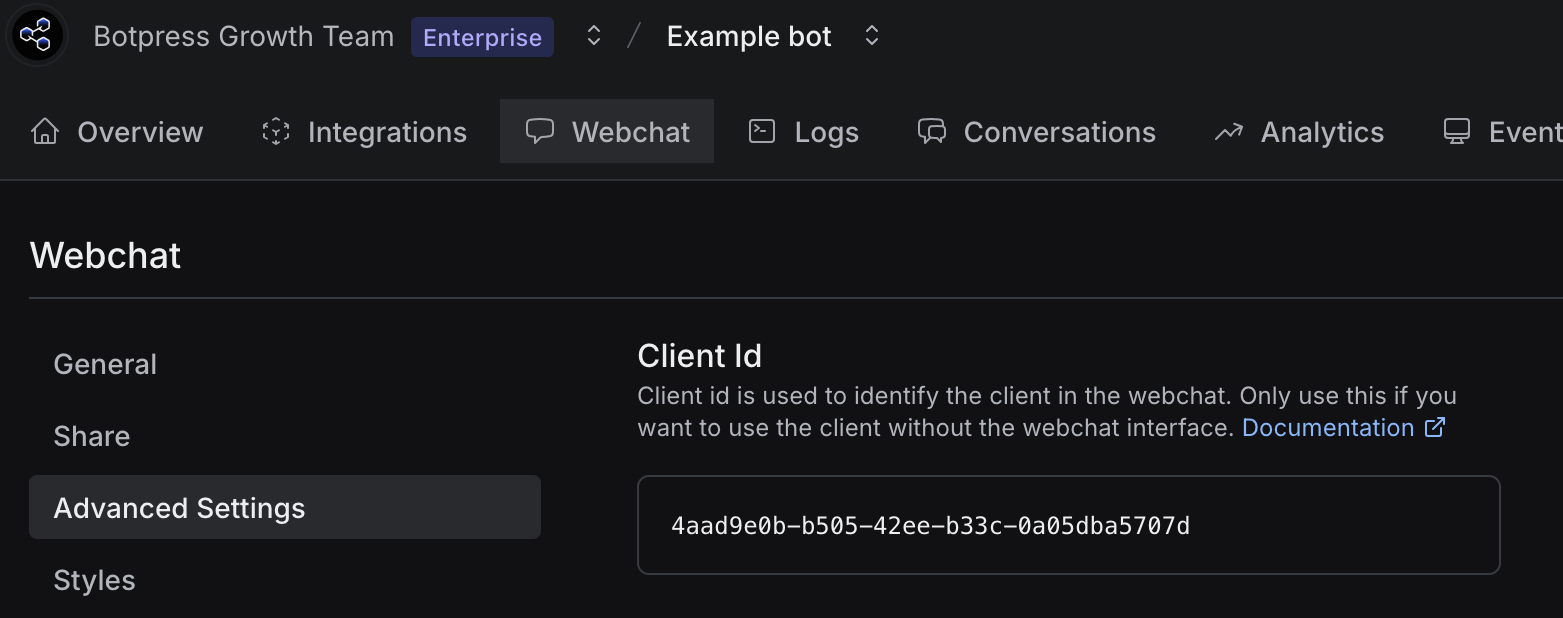
3. Add the code
import { getClient } from '@botpress/webchat'
//Add your Client ID here ⬇️
const clientId = "YOUR_CLIENT_ID";
const client = getClient({ clientId })
client.on("message", (message) => {
console.log("Received message", message); // Messages from the bot
});
await client.connect(); // Initialize the client
await client.sendMessage("Hello, Botpress!"); // Send a message to the bot
API Reference
The Webchat Client provides a set of properties and methods that allow you to manage conversations, send messages, and handle user sessions.
These are the available properties and methods:
Name | Description |
---|---|
mode | Defines the mode of the webchat client. 'messaging' for standard chat, and 'pushpin' for pinning messages or conversations. |
clientId | A unique identifier for the client, used to associate the client with a specific bot or session. |
apiUrl | The URL of the API that the webchat client will connect to. |
userId | The unique identifier of the user, if available. |
conversationId | The unique identifier of the current conversation, if available. |
on | A method to listen for specific events emitted by the webchat client. |
connect | Connects the client to the server with optional user credentials and data. |
disconnect | Disconnects the client from the server. |
getUser | Retrieves the current user's information. |
updateUser | Updates the current user's information. |
sendMessage | Sends a text message to the current conversation. |
sendFile | Sends a file to the current conversation and returns its details. |
sendEvent | Sends a custom event to the current conversation. |
switchConversation | Switches to a different conversation by its ID. |
conversationExists | Checks if a conversation with the specified ID exists. |
newConversation | Starts a new conversation. |
listMessages | Retrieves a list of messages from the current conversation. |
Live Demo
Updated 3 months ago